Check If Viewcontroller is Visible: Ultimate Guide
To check if a ViewController is visible, you can use the view’s window property which will be non-nil if the view is currently visible. Simply check the main view in the view controller to determine its visibility.
By doing so, you can avoid unnecessary loading of the view if it is already loaded. This method can be useful in scenarios where you need to perform certain actions only when the ViewController is visible.
Key Concepts In Viewcontroller Lifecycle
In iOS development, understanding the lifecycle of a ViewController is crucial for building robust and responsive applications. The ViewController lifecycle refers to the sequence of events that occur during the creation, presentation, and dismissal of a ViewController.
Ios Viewcontroller Hierarchy
When dealing with ViewControllers in iOS, it’s important to understand the hierarchy in which they operate. ViewControllers are organized in a hierarchical structure, with the root ViewController being the top-level view controller in an application’s view controller stack. This hierarchy allows for managing different screens and their interactions within an app.
The visibility of a ViewController plays a vital role in determining its behavior and responsiveness. By being aware of whether a ViewController is currently visible or not, you can take appropriate actions such as updating data, fetching new content, or pausing certain processes to optimize performance and improve the overall user experience.
The Lifecycle Events Affecting Visibility
The visibility of a ViewController is influenced by various lifecycle events. Here are some of the key events that affect ViewController visibility:
- ViewDidLoad: This event occurs when the ViewController’s view is loaded into memory. At this stage, the ViewController’s view hierarchy is constructed, but it may not be visible yet.
- ViewWillAppear: This event is triggered right before the ViewController’s view is about to become visible on the screen. It allows you to perform any necessary setup or updates before the view appears.
- ViewDidAppear: This event is fired when the ViewController’s view has appeared on the screen. It signifies that the view is fully visible and interactive, enabling you to initiate any actions that require user interaction.
- ViewWillDisappear: This event occurs when the ViewController’s view is about to be removed from the screen. It provides an opportunity to clean up resources or save any necessary data before the view disappears.
- ViewDidDisappear: This event is triggered once the ViewController’s view has completely disappeared from the screen. It indicates that the view is no longer visible and allows you to perform any final tasks or release any unused resources.
By understanding these lifecycle events, you can effectively determine the visibility of a ViewController and execute specific actions accordingly.
Remember to optimize your code by checking if a ViewController’s view is already loaded before invoking it, as unnecessary loading can impact performance. To do so, you can utilize the non-nil window property of the ViewController’s view to check its visibility status.
Being mindful of the ViewController lifecycle and visibility ensures a smoother navigation experience, improved memory management, and enhanced user engagement within your iOS applications.
`viewwillappear` And `viewdidappear`
To check if a `UIViewController` is currently visible, you can use the `view. window` property. If it is non-nil, then the view is currently visible. You can also check the main view in the view controller for visibility. This can be done by inspecting the view hierarchy or using code snippets available on platforms like GitHub and Stack Overflow.
Explanation Of Methods
When working with iOS development, there are two important methods that can be used to check the visibility of a `UIViewController`: `viewWillAppear` and `viewDidAppear`. The `viewWillAppear` method is called just before the view controller’s view is about to be added to the view hierarchy. It is typically used to prepare the view for display, such as updating data or refreshing the UI. This method is called every time the view is about to appear, even if the view is already visible, making it a good place to perform visibility checks. On the other hand, the `viewDidAppear` method is called when the view has fully transitioned onto the screen and is now visible to the user. This is the ideal place to perform tasks that rely on the visibility of the view, such as starting animations, loading additional data, or updating the UI based on the current state.Proper Use-cases For Visibility Checks
It’s important to understand when and why visibility checks are necessary in order to properly implement them in your iOS app. Here are some proper use-cases for visibility checks of a `UIViewController`: 1. Updating data: If you have a view that displays dynamic data, such as a news feed or a chat screen, you may want to update the data every time the view controller becomes visible. By performing a visibility check in the `viewWillAppear` method, you can trigger the data update only when the view is about to appear on the screen. 2. Animations: If you have animations in your app that should start or stop based on the view’s visibility, you can use visibility checks in the `viewDidAppear` method. For example, you may want to start playing a video animation when the view appears and pause it when the view disappears. 3. Resource management: In some cases, you may need to manage system resources or perform cleanup tasks when a view controller becomes visible or invisible. By using visibility checks in the appropriate methods, you can ensure that the necessary resources are allocated or deallocated at the right time. 4. Analytics tracking: Visibility checks can also be useful for tracking user engagement and behavior in your app. By tracking when a view becomes visible or invisible, you can gather data on how often users interact with specific screens or features. Overall, proper use of visibility checks can enhance the user experience, optimize resource usage, and provide valuable insights into user behavior. By utilizing the `viewWillAppear` and `viewDidAppear` methods, you can ensure that your app performs the necessary actions when a `UIViewController` becomes visible to the user.Benefits of visibility checks | Examples |
---|---|
|
|
if self.view.window != nil {
// The view is currently visible
// Perform necessary actions here
} else {
// The view is currently not visible
// Handle the visibility change accordingly
}
Accessing The `isviewloaded` Property
UnderstandingisViewLoaded
The isViewLoaded
property is a boolean value that indicates whether the view of a UIViewController
instance is currently loaded into memory. It can be used to determine if a view controller’s view is visible on the screen or not.
isViewLoaded
to verify visibilityWhen checking if a view controller’s view is visible, it is important to use the isViewLoaded
property in a safe and efficient manner. Here’s how you can do it:
- Step 1: Check if the view controller’s view is loaded
- Step 2: Verify visibility using the window property
- Step 3: Perform actions based on visibility status
Before checking the visibility of the view, it is necessary to verify if the view is loaded into memory. This can be done by checking the value of the isViewLoaded
property. If it returns true
, it means the view is loaded, and if it returns false
, it means the view is not yet loaded.
To determine if the view controller’s view is currently visible on the screen, you can check the window
property of the view. If the window
property is non-nil, it indicates that the view is currently visible. On the other hand, if the window
property is nil, it means the view is not currently visible.
Once you have determined the visibility status of the view controller’s view, you can perform specific actions accordingly. For example, if the view is visible, you can update its content or trigger certain animations. If the view is not visible, you can delay or cancel any unnecessary operations to optimize performance.
By following these steps and using the isViewLoaded
property effectively, you can ensure that you are accurately checking the visibility of a view controller’s view without unnecessary method invocations or performance overhead.
Utilizing The `window` Property
To check if a view controller is visible, you can utilize the `window` property of the view. If the view’s `window` property is non-nil, it means the view is currently visible. This can be done by checking the main view in the view controller.
The Role Of `window` In Viewcontroller
The `window` property plays a crucial role in determining whether a `ViewController` is currently visible or not. By checking the `window` property of a view, we can determine if the view is currently displayed on screen. The `window` property is non-nil if the view is visible and loaded in the view hierarchy. This allows us to perform checks and make decisions based on the visibility of the view.
How To Perform Checks Using `window`
To check if a `ViewController` is currently visible, we need to inspect the `window` property of the view. Here are the steps to perform the check:
- Access the main view of the `ViewController`. This can be done by referring to the root view of the `ViewController`.
- Check the `window` property of the main view. If the `window` property is non-nil, it means that the view is currently visible on screen.
- Based on the result of the check, we can perform actions or make decisions accordingly.
Performing the check using the `window` property is a more efficient way to determine the visibility of a `ViewController` compared to invoking the `view` method, which would load the view if it is not already loaded. By utilizing the `window` property, we avoid unnecessary loading of the view, making our code more optimized.
Observing View Hierarchies
To check if a UIViewController is currently visible, you can inspect the main view in the view controller. If the view’s window property is non-nil, it means the view is currently visible. This can be done by checking if the view is already loaded, avoiding unnecessary invocation of the view method.
Observing View Hierarchies Detecting a view within the active window To check if a UIViewController’s view is visible, you can utilize the view’s window property. If the window property is non-nil, it means that the view is currently visible. This allows you to detect whether a view controller’s view is being presented on the screen. Practical examples of hierarchy observation When it comes to observing view hierarchies, there are multiple ways to determine the visibility status of a view controller’s view. Here are a few practical examples: 1. Checking the main view in the view controller: “`swift if let window = view.window { // View is visible } else { // View is not currently visible } “` 2. Finding the top visible view controller in a UINavigationController: “`swift if let topViewController = navigation.visibleViewController?.topMostViewController() { // Top view controller is visible } else { // Top view controller is not currently visible } “` 3. Checking the visibility status of a view in an iOS app: “`swift if let window = UIApplication.shared.windows.first(where: { $0.isKeyWindow }), let rootViewController = window.rootViewController, rootViewController.view.window != nil { // View is visible } else { // View is not currently visible } “` By observing the view hierarchy using these examples, you can efficiently determine whether a view controller’s view is visible or not. This provides valuable insights for performing specific actions based on the visibility status. In conclusion, by simply checking the window property of the view, you can effectively detect whether a view controller’s view is currently visible. This allows you to make informed decisions and perform relevant actions based on the visibility status of the view.Handling Navigation Controllers
Navigation controllers are a crucial part of building user-friendly and intuitive iOS apps. They allow users to navigate seamlessly between different screens and view controllers. However, when it comes to managing the visibility of view controllers within a navigation stack, things can get a bit tricky. In this section, we will explore how to check if a view controller is visible within a navigation controller and ensure smooth handling of visibility.
Managing Visibility In A Stack Of Viewcontrollers
When dealing with a stack of view controllers within a navigation controller, it becomes essential to determine whether a particular view controller is currently visible to the user. Luckily, there is a simple way to achieve this using the `view.window` property in iOS.
The `view.window` property of a view controller is non-nil if the view is currently visible. This means that by checking the main view of the view controller, we can determine its visibility. However, it is important to note that invoking the `view` method to load the view can be unnecessary and undesirable in certain scenarios.
To optimize the process, we should first check if the view is already loaded before determining its visibility. By inspecting the main view of the view controller, we can avoid unnecessary loading of views and improve performance.
Let’s take a look at a code snippet that demonstrates how to check if a UIViewController’s view is currently visible:
if let mainView = viewController.viewIfLoaded, mainView.window != nil {
// View controller is currently visible
} else {
// View controller is not currently visible
}
In the above code, we first check if the view is loaded using the `viewIfLoaded` property. If the view is loaded and the `window` property is non-nil, it means that the view controller is currently visible in the navigation stack.
By utilizing this approach, we can efficiently manage the visibility of view controllers within a navigation controller and ensure a seamless user experience.
For further reference and additional implementations of this feature, you can explore the relevant information available on Stack Overflow or GitHub Gist.
Dealing With Tabbar Controllers
TabBar Controllers are a commonly used component in iOS app development, allowing users to navigate between different sections or functionalities of the app. When working with TabBar Controllers, it’s important to be able to check if a specific ViewController is currently visible. This can be useful for various purposes, such as updating the UI or performing specific actions when a certain ViewController is active. In this section, we will explore different approaches to recognize visible ViewControllers in a tab-based application.
Recognizing Visible Viewcontroller In A Tab-based Application
In a tab-based application, each tab represents a different ViewController. To check if a specific ViewController is currently visible, you can utilize the view’s window property. If the view is currently visible, the window property will be non-nil. Here’s an example of how you can implement this:
if let currentViewController = tabBarController.selectedViewController, currentViewController.view.window != nil {
// The current ViewController is visible
// Perform necessary actions or updates here
} else {
// The current ViewController is not visible
}
By accessing the selectedViewController property of the TabBar Controller, you can obtain the currently selected ViewController. Checking if its view’s window property is non-nil determines if it is currently visible. You can tailor your application logic based on whether the ViewController is visible or not.
By following this approach, you can ensure that your app responds accurately based on the visibility of specific ViewControllers within the TabBar Controller.
Avoid Unintended View Loading
When developing an iOS application, it is important to ensure that the view controller is only loaded and visible when necessary. Unintended view loading can lead to performance issues and unnecessary resource consumption. Therefore, it is crucial to implement proper techniques to prevent unnecessary loading and ensure efficient visibility checks.
Techniques To Prevent Unnecessary Loading
To avoid unintended view loading, there are several techniques that you can implement:
- Check the main view in the view controller: Before invoking the view method, examine the main view of the view controller to determine if it is already loaded. You can do this by checking if the view’s window property is non-nil. If it is non-nil, it indicates that the view is currently visible, and there is no need to load it again.
- Inspect the visibility status of the view controller: By analyzing the visibility status of the view controller, you can determine whether the view is currently visible or not. This can be done by examining the view’s window property as discussed above.
Best Practices For Efficient Visibility Checks
To ensure efficient visibility checks, consider the following best practices:
- Avoid invoking the view method unnecessarily: Before loading the view, always check if it is already loaded. Invoking the view method when the view is already loaded can cause unnecessary overhead. By inspecting the main view of the view controller, you can determine if the view needs to be loaded or not.
- Use the viewWill/DidAppear() methods: These methods can be utilized to determine if a view controller’s view is currently visible. Override either the viewDidAppear() or viewDidDisappear() method, depending on your requirements, to perform visibility checks.
- Consider utilizing view containment: If you are working with complex view hierarchies or view controller transitions, it may be beneficial to use view containment. By using child view controllers and observing their visibility, you can optimize your visibility checks and improve overall performance.
By implementing these techniques and following best practices, you can ensure that your view controllers are only loaded and visible when necessary, thereby optimizing resource usage and enhancing the overall performance of your iOS application.
Ensuring Accuracy In Complex Layouts
To check if a UIViewController is visible, you can use the view’s window property. If the property is non-nil, then the view is currently visible. You can check the main view in the view controller to determine its visibility. Make sure to avoid unnecessary loading of the view by checking if it is already loaded.
When working with complex layouts in iOS development, it is crucial to accurately determine the visibility of a ViewController. This ensures that the appropriate actions are taken based on the current state of the view. In this article, we will explore strategies and tips to ensure accurate visibility determination in complex ViewController structures.
Strategies For Complex Viewcontroller Structures
When dealing with complex ViewController structures, it is important to have a clear understanding of the hierarchy and relationships between the different ViewControllers. Here are some strategies to consider:
- Identify the root ViewController: In cases where multiple ViewControllers are presented on top of each other, it is essential to identify the root ViewController. This can be achieved by traversing through the hierarchy of presented ViewControllers until reaching the topmost ViewController.
- Use the UINavigationController: If your ViewController is embedded in a UINavigationController, you can check the visibility by accessing the visibleViewController property. This returns the currently visible ViewController.
- Inspect the UITabBarController: If your ViewController is a part of a UITabBarController, you can determine its visibility by examining the currently selected tab. The ViewController associated with the selected tab is the visible ViewController.
Tips For Accurate Visibility Determination
Ensuring accurate visibility determination is crucial in complex layouts. Here are some tips to help you achieve this:
- Avoid unnecessary view loading: Invoking the view method of a ViewController causes the view to load, which may not be necessary. Before checking the visibility, verify if the view is already loaded to optimize performance and avoid undesirable side effects.
- Check the window property: The window property of a view is non-nil if the view is currently visible. By checking the main view in the ViewController and inspecting its window property, you can accurately determine if the ViewController is visible.
- Handle ViewController transitions: When presenting or dismissing ViewControllers, ensure to update the visibility status accordingly. Presenting a new ViewController replaces the current content, while dismissing a ViewController changes the visibility status of its content.
By following these strategies and tips, you can ensure accurate visibility determination in complex ViewController structures. This allows you to take the appropriate actions based on the current state of the view, providing a seamless user experience.
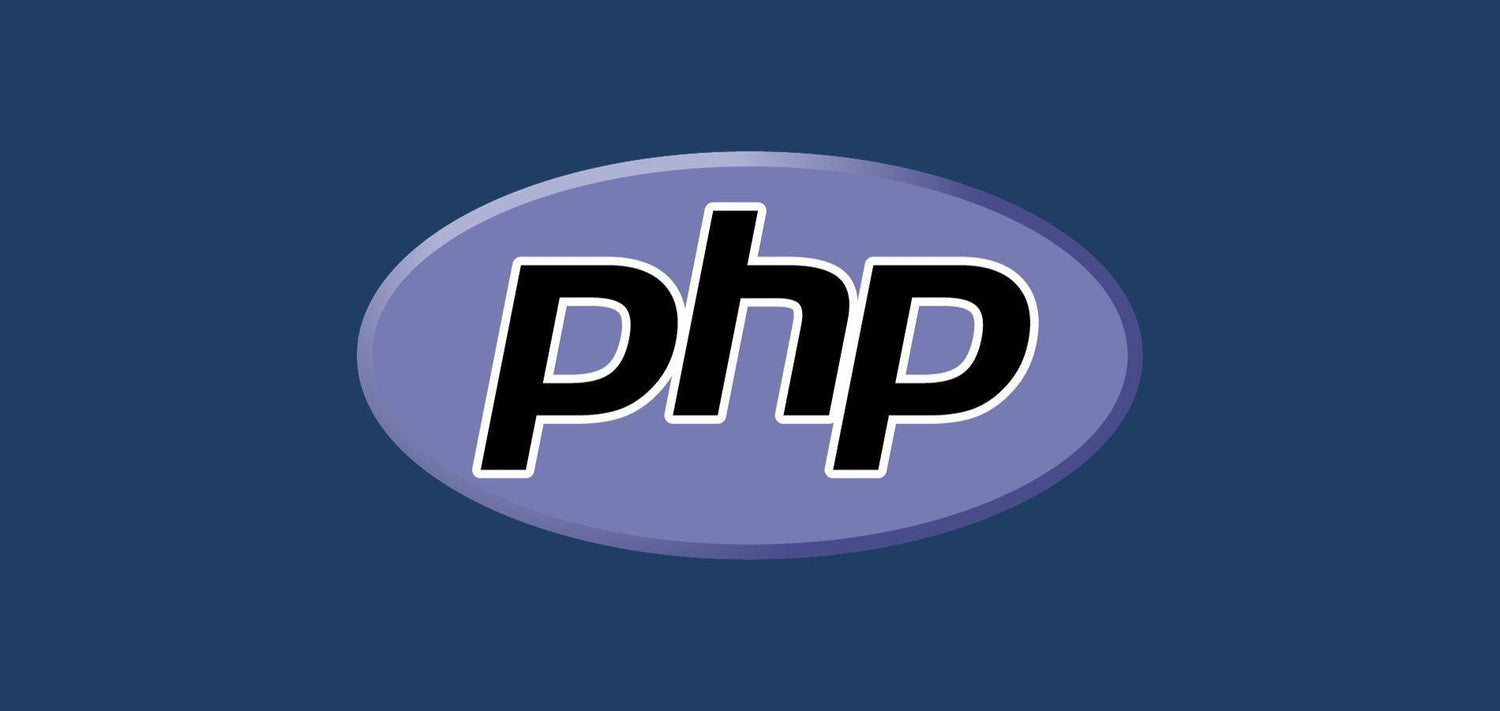
Credit: www.coderchamp.com
Frequently Asked Questions Of How To Check If Viewcontroller Is Visible
How To Check Viewcontroller Class In Swift?
To check if a ViewController class is visible in Swift, you can check the view’s window property. If it is non-nil, the view is currently visible. To do this, check the main view in the view controller without invoking the view method unnecessarily.
You can find more information on how to achieve this on resources such as Stack Overflow and GitHub Gist.
Is A Viewcontroller A View?
A ViewController is not a view itself, but it manages a single root view and can contain multiple subviews.
How Do You Add Views To Viewcontroller?
To add views to a UIViewController, you can drag and drop them onto the view controller scene in storyboards. The main view of the view controller can be accessed using the view property. Make sure to check if the view is already loaded before adding any additional views.
Keep in mind that a UIViewController manages a single root view which can contain multiple subviews.
What Is A Uiviewcontroller?
A UIViewController is a class in iOS development that manages a single “view” in the app’s user interface. It controls the user interactions and coordinates with other objects in the app. The visibility of a UIViewController’s view can be checked by examining its window property, which is non-nil if the view is currently visible.
By checking the main view in the view controller, you can determine if it is already loaded and visible.
Conclusion
To determine if a ViewController is visible, you can check the view’s window property. If it is non-nil, the view is currently visible. However, it’s recommended to first check if the view is already loaded to avoid unnecessary loading. This can be done by inspecting the main view in the ViewController.
By following these steps, you can easily determine the visibility status of a ViewController. Implementing these techniques will help you efficiently check if a ViewController is visible without unnecessary loading.